#importar las librerias.
import pygame
import sys
# Inicializar pygame
pygame.init()
# Definir colores
NEGRO = (0, 0, 0)
BLANCO = (255, 255, 255)
# Definir dimensiones de la ventana
ancho_ventana = 800
alto_ventana = 600
dimensiones_ventana = (ancho_ventana, alto_ventana)
# Crear ventana
ventana = pygame.display.set_mode(dimensiones_ventana)
pygame.display.set_caption("Pac-Man")
# Variables del juego
posicion_x = 200
posicion_y = 200
# Bucle principal del juego
while True:
for evento in pygame.event.get():
if evento.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Lógica del juego
# Actualizar pantalla
ventana.fill(NEGRO)
pygame.draw.circle(ventana, BLANCO, (posicion_x, posicion_y), 10)
pygame.display.flip()
Este es solo un ejemplo básico que muestra un círculo blanco en movimiento dentro de una ventana negra. Tendrías que agregar la lógica adicional para implementar las reglas del juego de Pac-Man, como el movimiento del personaje, las colisiones con los enemigos y los puntos, entre otras cosas. Además, puedes añadir imágenes, sonidos y otros elementos visuales para mejorar la apariencia del juego.
Recuerda que este código utiliza la biblioteca Pygame, por lo que deberás tenerla instalada en tu entorno de Python para ejecutar este código correctamente.
Reto #1 Hacer un pacman con Python, verifique el código agregue lo siguiente:
1. Cambia el tablero.
2. Cambia el número de fantasmas.
3. Cambia el lugar donde comienza pacman.
4. Haz que los fantasmas sean más rápidos o más lentos.
5. Haz que los fantasmas sean más inteligentes. """ #Pacman arcade viejo:
from random import choice
from turtle import *
from freegames import floor, vector
state = {'score': 0}
path = Turtle(visible=False)
writer = Turtle(visible=False)
aim = vector(5, 0)
pacman = vector(-40, -80)
ghosts = [[vector(-180, 160), vector(5, 0)],[vector(-180, -160), vector(0, 5)],[vector(100, 160), vector(0, -5)],[vector(100, -160), vector(-5, 0)],
]
# fmt: apagado
tiles = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0,0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0,0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0,0, 1, 0, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0,0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0,0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0,0, 1, 0, 0, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0,0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0,0, 0, 0, 0, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0,0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0,0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0,0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0,0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 0, 0, 0,0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 0,0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0,0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0,0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0,0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
]
def square(x, y):
"""Draw square using path at (x, y)."""
path.up()
path.goto(x, y)
path.down()
path.begin_fill()
for count in range(4):
path.forward(20)
path.left(90)
path.end_fill()
def offset(point):
"""Return offset of point in tiles."""
x = (floor(point.x, 20) + 200) / 20
y = (180 - floor(point.y, 20)) / 20
index = int(x + y * 20)return index
def valid(point):
"""Return True if point is valid in tiles."""
index = offset(point)
if tiles[index] == 0:
return False
index = offset(point + 19)
if tiles[index] == 0:
return False
return point.x % 20 == 0 or point.y % 20 == 0
def move():
"""Move pacman and all ghosts."""
writer.undo()
writer.write(state['score'])
clear()
if valid(pacman + aim):
pacman.move(aim)
index = offset(pacman)
if tiles[index] == 1:
tiles[index] = 2
state['score'] += 1
x = (index % 20) * 20 - 200
y = 180 - (index // 20) * 20
square(x, y)
up()
goto(pacman.x + 10, pacman.y + 10)
dot(20, 'yellow')
for point, course in ghosts:
if valid(point + course):
point.move(course)
else:
options = [
vector(5, 0),
vector(-5, 0),
vector(0, 5),
vector(0, -5),
]
plan = choice(options)
course.x = plan.x
course.y = plan.y
up()
goto(point.x + 10, point.y + 10)
dot(20, 'red')
update()
for point, course in ghosts:
if abs(pacman - point) < 20:
return
ontimer(move, 100)
def change(x, y):
"""Change pacman aim if valid."""
if valid(pacman + vector(x, y)):
aim.x = x
aim.y = y
Imagenes continuación:
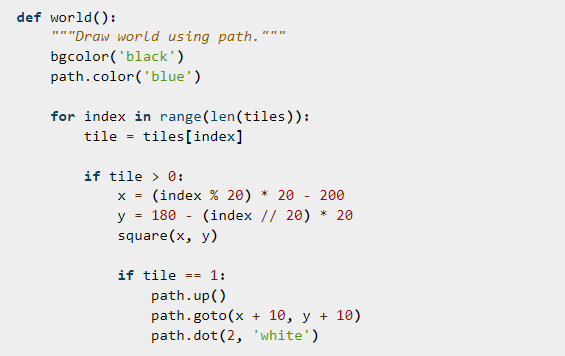
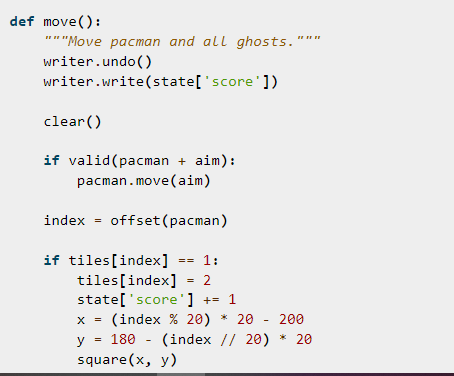
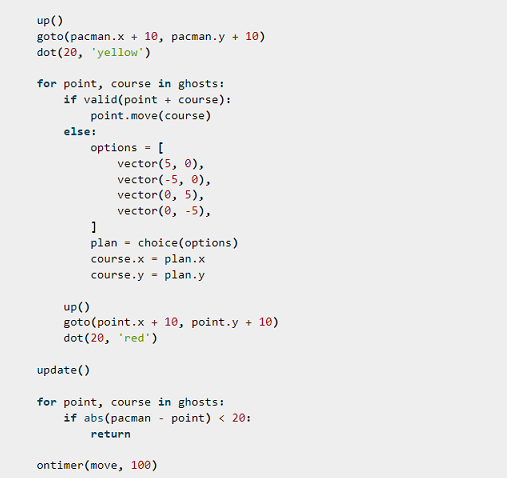
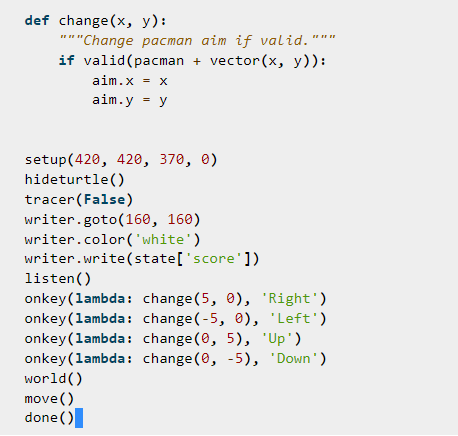
Comments